Bing Rewards Auto Searcher With Python 3
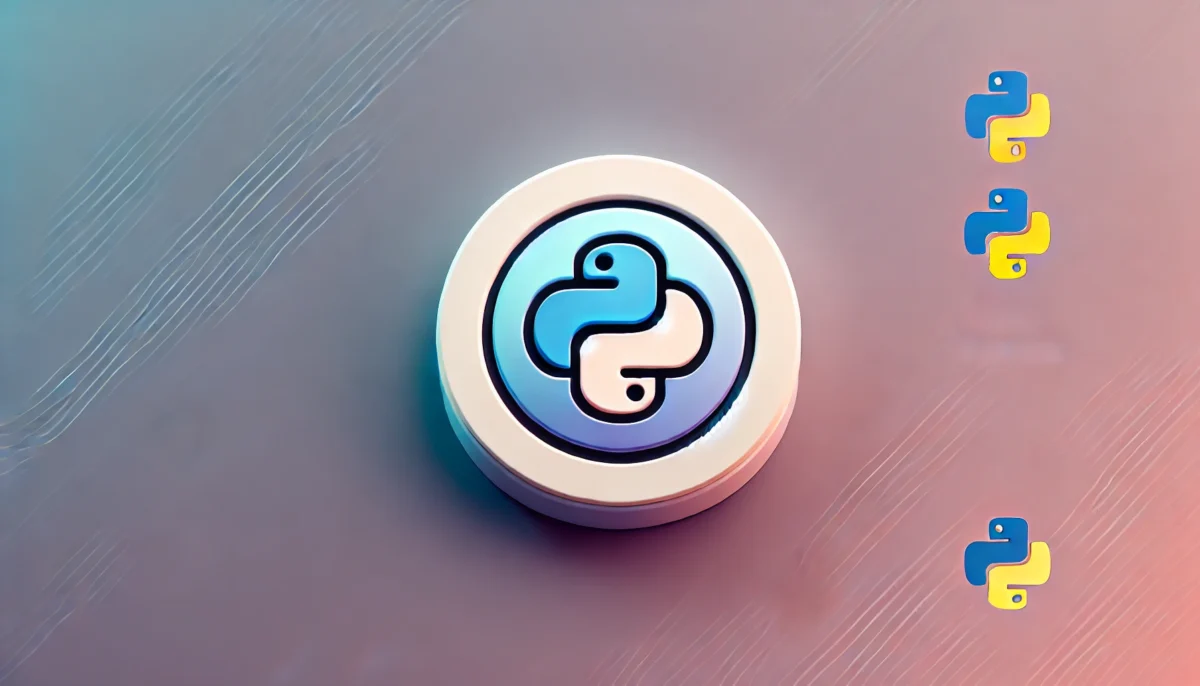
This Bing Rewards auto searcher simple Python 3 script will automatically call your default browser, open Bing and then perform 30 random searches. This script has only been tested with Windows 10 but should be able to work on Linux and MacOS.
Install Python 3.7
If you haven’t already you will need to have Python 3.7 installed. You can check out my article here for Windows 10 and Centos 7.5.
Install Required Modules
We will be using some 3rd party modules in our script so we need to use pip to install them. In Windows PowerShell run the following commands:
pip3 install pyautogui
pip3 install requests
Bing Rewards Auto Searcher Script
Below is the Python script. Simply save this file wherever you like with a .py extension. You will need to adjust file paths and create any files referenced below.
import pyautogui
import time
import webbrowser
import requests
import random
def Diff(li1, li2):
return (list(set(li1) - set(li2)))
url = 'https://www.bing.com/'
word_site = "https://www.myhelpfulguides.com/keywords.txt"
response = requests.get(word_site)
words = response.text.splitlines()
for i in range(30):
f = open('D:/Documents/Python Scripts/bingSearch/usedKeywords.txt', 'r')
used = f.read().splitlines()
new = Diff(words, used)
search = random.choice(new)
f = open('D:/Documents/Python Scripts/bingSearch/usedKeywords.txt', 'a')
f.write(search + "\n")
f.close()
webbrowser.open_new_tab(url)
sleep = random.randint(3,36)
time.sleep(sleep)
pyautogui.typewrite(search, interval=0.01)
pyautogui.typewrite('\n', interval=0.1)
pyautogui.hotkey('ctrl', 'w')
print(f"The count is {i} with a pause of {sleep} seconds between searches. We searched for {search}")
Feel free to adjust the code as you see fit. It is pretty easy to follow. If you want to change how many searches are performed then change:
for i in range(30):
Where 30 is the amount of searches done.
If you want to adjust the sleep time then edit:
sleep = random.randint(3,36)
Where 3 and 36 are seconds and the function will randomly pick a number between those two numbers.
You will obviously want to make sure that you are logged in to your Microsoft account and cookied to Remember so that you get credit for the searches.
Disclaimer
Automation of searches for Bing Rewards is against the Bing Rewards ToS. Use at your own risk!
Bing searches using Python! It’s simple, customizable, and well-explained. Just be cautious with Bing Rewards’ ToS. Overall, a helpful guide for beginners.