Create A Google Trends Keyword Scraper In Python 2024
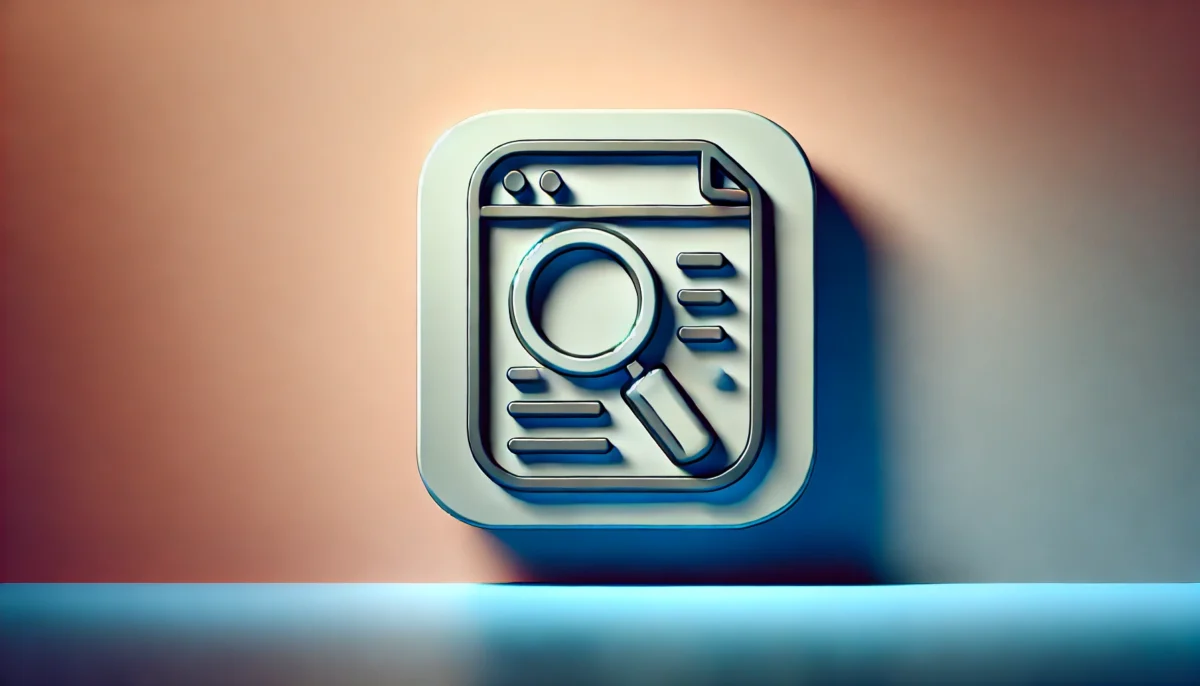
For my Bing Rewards Auto Searcher python script I was using a word list and randomly combining words to create phrases. This worked fine but I worried that the searches would be flagged by Bing because, well, they made no sense.
So I looked for a way to easily gather a list of legitimate search phrases and there is no better place to get that thanĀ Google Trends. Google is nice and provides an RSS feed of their daily search trends so I went about creating a Google Trends keyword scraper that would parse that list and create a simple text file of each keyword that ends up trending. Below is the script.
All of my code is run on Python 3.7. If you need help on installing Python you can check out my Windows 10 and Centos 7.5 installation guides.
Google Trends Keyword Scraper In Python
The below script is pretty simple. Just copy and run, making sure that you create all the needed files and adjust the locations used below. You can do many things with this but I simply need it to create a txt file with a rolling list of the keywords.
import codecs
import os
import urllib.request
from xml.etree import ElementTree
url = 'https://trends.google.com/trends/trendingsearches/daily/rss?geo=FR'
keywords_filename = './keywords.txt'
tmp_filename = './keywords_tmp.xml'
urllib.request.urlretrieve(url, tmp_filename)
if os.path.isfile(keywords_filename):
with open(keywords_filename, 'r') as f:
old_list = f.read().splitlines()
else:
old_list = []
tree = ElementTree.parse(tmp_filename)
root = tree.getroot()
fresh_list = [title.text.lower()
for title in root.iter('title')]
try:
fresh_list.remove('daily search trends')
except ValueError:
pass
output = '\n'.join(a for a in list(set(old_list + fresh_list)))
with codecs.open('./keywords.txt', 'w+', "utf-8") as f:
f.write(output)
os.remove(tmp_filename)
Live Example
I have made my list public so that anyone that wishes to use it can. You can check it out at https://myhelpfulguides.com/keywords.txt. The script that powers my list runs every 5 minutes so it should be very up to date.